How to Show Date Events and Holiday in ASP.Net Calendar Control - Microsoft ASP Solutions
Calendar is an asp.net web server control. this web server control allow us to select an individual date or date range at a time. calendar control is rendered as an html table and each day of calendar control contains a LinkButton control that raises an event when it is clicked.
calendar control have many built in properties to set or change its day cells text color, background color, border style etc. but calendar control have no property to change its individual day cell or any specific day cell styles programmatically.
calendar DayRender event occurs when each day is created in the control hierarchy of the calendar control. so it is possible to change the style of any individual day cell in calendar control before it display in web browser. by using this event we can modify the appearance of specific day cell or cells before it display in web page.
calendar DayRender event allow us to attach a css class for any specific day cell in calendar control. by this way we can apply core css style to specified day cells in calendar control. in this example code we applied a mouse hover effect for specific day cells of calendar control.
the following asp.net c# example code demonstrate us how can we customize the appearance of specified day cells in calendar control by attaching css class to those day cells in an asp.net application.
Design Page Code Below
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Calender.aspx.cs" Inherits="Calender" %> <!DOCTYPE html> <html xmlns="http://www.w3.org/1999/xhtml"> <head runat="server"> <title></title> <meta charset="utf-8" /> <meta name="viewport" content="width=device-width, initial-scale=1" /> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/3.3.7/css/bootstrap.min.css" /> <style> .isOtherMonth { background-color: transparent !important; } .isOtherMonth table td span { display:none; } table tr td { vertical-align:top; } table tr td a { float:right; padding:10px; font-size:18px; } table tr td span { } .table-bordered { border: 1px solid #080000; } </style> </head> <body> <form id="form1" runat="server"> <div> <asp:DropDownList runat="server" ID="DrpMonth" > <asp:ListItem Value="1">January</asp:ListItem> <asp:ListItem Value="2">February</asp:ListItem> <asp:ListItem Value="3">March</asp:ListItem> <asp:ListItem Value="4">April</asp:ListItem> <asp:ListItem Value="5">May</asp:ListItem> <asp:ListItem Value="6">June</asp:ListItem> <asp:ListItem Value="7">July</asp:ListItem> <asp:ListItem Value="8">August</asp:ListItem> <asp:ListItem Value="9">September</asp:ListItem> <asp:ListItem Value="10">October</asp:ListItem> <asp:ListItem Value="11">November</asp:ListItem> <asp:ListItem Value="12">Decemeber</asp:ListItem> </asp:DropDownList> <asp:Button runat="server" ID="btnChange" Text="Submit" OnClick="btnChange_Click"/> <br /><br /><br /> <asp:Calendar runat="server" ID="Calendar1" FirstDayOfWeek="Monday" BorderWidth="0" OnDayRender="Calendar1_DayRender" ShowGridLines="false" ShowTitle="false" > <SelectedDayStyle Width="100px" CssClass="table table-responsive" Height="100px" /> <DayHeaderStyle BorderWidth="0" Font-Size="X-Large" CssClass="text-center" ForeColor="Brown" Width="100px" /> <DayStyle Width="100px" Height="100px" CssClass="table table-bordered table-responsive" /> </asp:Calendar> </div> </form> </body> </html>
Code Page Coding Below
using System; using System.Collections.Generic; using System.Data; using System.Linq; using System.Web; using System.Web.UI; using System.Web.UI.WebControls; public partial class Calender : System.Web.UI.Page { protected void Page_Load(object sender, EventArgs e) { } protected void btnChange_Click(object sender, EventArgs e) { int M = Convert.ToInt32(DrpMonth.SelectedValue); Calendar1.TodaysDate = new DateTime(2018, M , 01); } protected void Calendar1_DayRender(object sender, DayRenderEventArgs e) { // Change the background color of the days in the month // to yellow. if (e.Day.IsOtherMonth) { e.Cell.Text = String.Empty; e.Cell.Height = 0; e.Cell.CssClass = "isOtherMonth"; } // Add custom text to cell in the Calendar control. if (e.Day.Date.Month == 06) { if (e.Day.Date.Day == 05) { e.Cell.Controls.Add(new LiteralControl("<table style='height:50px;width:100%;'><tr><td style='vertical-align:bottom;padding:5px;'><span class='label label-primary'>Holiday</span></td></tr></table>")); e.Cell.BackColor = System.Drawing.Color.Green; e.Cell.ForeColor = System.Drawing.Color.White; } } if (e.Day.Date.Month == 06) { if (e.Day.Date.Day == 10) { e.Cell.Controls.Add(new LiteralControl("<table style='height:50px;width:100%;'><tr><td style='vertical-align:bottom;padding:5px;'><span class='label label-primary'>Holiday</span></td></tr></table>")); e.Cell.BackColor = System.Drawing.Color.DarkSlateBlue; e.Cell.ForeColor = System.Drawing.Color.White; } } if (e.Day.Date.Month == 06) { if (e.Day.Date.Day == 07) { e.Cell.Controls.Add(new LiteralControl("<table style='height:50px;width:100%;'><tr><td style='vertical-align:bottom;padding:5px;'><span class='label label-primary'>Holiday</span></td></tr></table>")); e.Cell.BackColor = System.Drawing.Color.DarkMagenta; e.Cell.ForeColor = System.Drawing.Color.White; } } if (e.Day.Date.Month == 06) { if (e.Day.Date.Day == 30) { e.Cell.Controls.Add(new LiteralControl("<table style='height:50px;width:100%;'><tr><td style='vertical-align:bottom;padding:5px;'><span class='label label-primary'>Holiday</span></td></tr></table>")); e.Cell.BackColor = System.Drawing.Color.DarkOrchid; e.Cell.ForeColor = System.Drawing.Color.White; } } if (e.Day.Date.Month == 06) { if (e.Day.Date.Day == 22) { e.Cell.Controls.Add(new LiteralControl("<table style='height:50px;width:100%;'><tr><td style='vertical-align:bottom;padding:5px;'><span class='label label-primary'>Holiday</span></td></tr></table>")); e.Cell.BackColor = System.Drawing.Color.DeepPink; e.Cell.ForeColor = System.Drawing.Color.White; } } } }
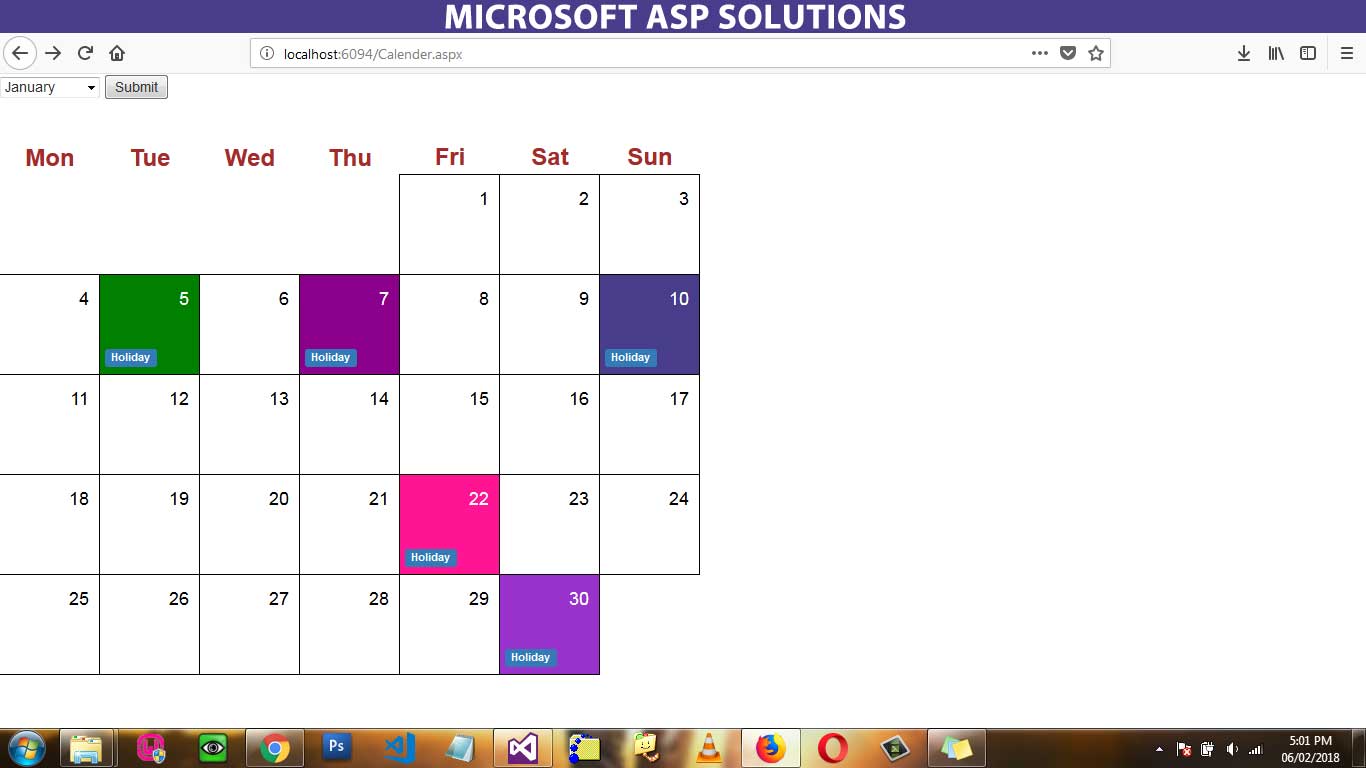
good knowledge
ReplyDeletehmm thnakq #mycommerceinfo
Deletevery useful for me
ReplyDeleteohhhhh great thanks #mycommerceinfo
Deletevery useful for me
ReplyDeleteevent
Thanks @Ali
DeleteWelcome to the BEST online casino site! - ChoGiocasino Casino
ReplyDeleteThe online casino site offers an assortment 메리트 카지노 도메인 of unique games and an 우리카지노 amazing casino The top casino 더킹 카지노 for slots 카지노 꽁 머니 is the 메리트 카지노 가입코드 Red Hot Megaways.